Apple Collector Project Tutorial With Using HTML CSS & JAVASCRIPT
In this article, we will explore the concept of an Apple Collector project developed using HTML, CSS, and JavaScript. This project aims to create a fun and interactive game where players collect apples by moving a basket across the screen. We will delve into the step-by-step process of building this project, starting from the design phase to the implementation of functionality using the mentioned web technologies.
Designing the User Interface
Before diving into the coding aspect, it’s crucial to design the user interface (UI) for our Apple Collector project. A visually appealing and intuitive UI enhances the overall user experience. Let’s outline the necessary design steps:
Choosing a Color Scheme
Selecting an appropriate color scheme is essential for creating an engaging interface. Considering the nature of the game, vibrant and cheerful colors would work well. Incorporating shades of green, red, and brown can provide a natural and playful ambiance.
Creating the Basket Element
The basket serves as the player’s tool for collecting apples. It should be visually distinct from the background and other elements. Adding realistic details, such as a woven texture, can enhance its appearance and make it more visually appealing.
Positioning the Apples
Strategically placing the apples on the screen will challenge the players to move the basket effectively. Randomizing the positions of the apples adds an element of surprise and increases replay value. Ensure that the apples are easily distinguishable from the background and basket.
Implementing HTML Structure
With the design phase complete, we can move on to implementing the HTML structure for our Apple Collector project. This step involves setting up the necessary HTML elements and styling them using CSS:
Setting up the Document Structure
Begin by creating the basic structure of the HTML document. Use appropriate HTML tags such as <html>
, <head>
, and <body>
. Consider using a <div>
element to contain the game elements.
Adding CSS Styling
Apply CSS styling to the HTML elements to achieve the desired visual effects. Use classes and IDs to target specific elements and define their appearance. Remember to keep the code organized and use proper indentation for readability.
Including JavaScript Dependencies
To enable interactivity and implement game logic, we need to include JavaScript dependencies. Utilize the <script>
tag to link the JavaScript file to the HTML document. Make sure to load the dependencies in the correct order.
Handling Interactivity with JavaScript
JavaScript plays a vital role in adding interactivity to our Apple Collector project. We will implement various functionalities using JavaScript to enhance the gameplay experience:
Capturing User Input
Capture user input, such as mouse movements or arrow key presses, to control the movement of the basket. Utilize event listeners to detect these interactions and trigger the appropriate actions.
Moving the Basket
Implement the logic for moving the basket based on user input. Update the position of the basket element according to the detected input, ensuring it stays within the game boundaries.
Collecting Apples
Detect collisions between the basket and the apples. When a collision occurs, update the score and remove the collected apple from the screen. Keep track of the number of apples collected to provide feedback to the player.
Enhancing the Gameplay Experience
To make our Apple Collector project more engaging, we can incorporate additional features that enhance the overall gameplay experience:
Adding Sound Effects
Implement sound effects for actions such as collecting apples or reaching a specific score milestone. These audio cues provide auditory feedback and contribute to the immersive experience.
Implementing Score Tracking
Track the player’s score throughout the game. Display the current score on the screen and update it whenever an apple is collected. Consider adding visual indicators for score milestones or high scores.
Setting Time Limits
Introduce a time limit to add a sense of urgency to the gameplay. Display a countdown timer and end the game when the time runs out. Provide feedback on the final score achieved within the given time.
Conclusion
In conclusion, building an Apple Collector project using HTML, CSS, and JavaScript allows us to create an interactive and enjoyable game where players collect apples using a moving basket. By following the steps outlined in this article, you can develop a unique and engaging project that showcases your web development skills.
FAQs
Q: Can I customize the appearance of the basket and apples?
- A: Yes, you can customize the visual aspects of the basket and apples to suit your preferences or project requirements.
Q: Is it possible to add more levels or challenges to the game?
- A: Absolutely! You can extend the Apple Collector project by introducing multiple levels, power-ups, or obstacles to increase the complexity and excitement.
Q: Can I deploy the game on a website or share it with others?
- A: Yes, once you have completed the project, you can deploy it on a website or share it with others by hosting it online.
Q: Do I need prior coding experience to create the Apple Collector project?
- A: While some familiarity with HTML, CSS, and JavaScript would be beneficial, this project is suitable for both beginners and those with coding experience.
Q: Are there any additional resources or tutorials available for further learning?
- A: Yes, there are numerous online resources and tutorials that can help you explore more advanced concepts and techniques related to web development.
By following this guide, you will be able to develop an engaging Apple Collector project using HTML, CSS, and JavaScript. Enjoy the process of creating a fun and interactive game while honing your web development skills. Happy coding!
Index.html
Apple Collector
- Time Left: 30
- Score: 0
Hi: 0
style.css
html,
body {
margin: 0;
padding: 0;
box-sizing: border-box;
height: 100vh;
/* background: linear-gradient(45deg, rgb(255, 165, 47), rgb(191, 30, 255)); */
/* background-color: #aaf; */
background: linear-gradient(45deg, rgb(255, 141, 47), dodgerblue);
font-family: "Sansita Swashed", cursive;
}
#scene {
width: 500px;
height: 500px;
/* background-color: #aaf; */
background: linear-gradient(45deg, rgb(255, 165, 47), rgb(191, 30, 255));
overflow: hidden;
margin: 16px auto;
position: relative;
box-shadow: rgba(0, 0, 0, 0.25) 0px 54px 55px, rgba(0, 0, 0, 0.12) 0px -12px 30px, rgba(0, 0, 0, 0.12) 0px 4px 6px, rgba(0, 0, 0, 0.17) 0px 12px 13px, rgba(0, 0, 0, 0.09) 0px -3px 5px;
}
#tree {
position: absolute;
display: block;
width: 300px;
bottom: 80px;
left: 100px;
}
#ground {
position: absolute;
display: block;
width: 500px;
bottom: 0;
}
.apple {
position: absolute;
top: 225px;
left: 120px;
width: 26px;
height: auto;
transition: top .5s ease;
}
#scoreboard {
width: 500px;
margin: 4px auto;
padding: 0;
background: linear-gradient(45deg, rgb(255, 165, 47), rgb(191, 30, 255));
font-family: "Sansita Swashed", cursive;
box-shadow: rgba(0, 0, 0, 0.25) 0px 54px 55px, rgba(0, 0, 0, 0.12) 0px -12px 30px, rgba(0, 0, 0, 0.12) 0px 4px 6px, rgba(0, 0, 0, 0.17) 0px 12px 13px, rgba(0, 0, 0, 0.09) 0px -3px 5px;
}
#scoreboard:after {
clear: both;
display: block;
content: " ";
}
#scoreboard li {
list-style: none;
box-sizing: border-box;
width: 33%;
padding: 4px;
text-align: center;
float: left;
}
span {
font-weight: bold;
color: rgb(96, 85, 255);
}
main.js
/**
* If you have completely understood whats going on here in the code then
* your next challenge is to recreate this game in Object Oriented Style.
* Also you can add some more effects like moving clouds, birds, sounds to game.
*/
//these are global variables because they need to be accessed by multiple functions.
var score = 0,
highScore = 0,
time = 30,
timer;
var IsPlaying = false;
var timeBoard = document.getElementById('time'),
scoreBoard = document.getElementById('score'),
btnStart = document.getElementById('btn');
/**
* Makes the provided element fall down by changing the top property.
* @param {HTMLElement} apple
*/
function fallDown(apple) {
if (!(IsPlaying && apple instanceof HTMLElement)) {
return;
}
//store the current top position for future refrence.
apple.setAttribute('data-top', apple.style.top);
//change the top position, this is animated using transition property in CSS
apple.style.top = "380px";
//increase score
score = score + 5;
//show the score by calling this function
renderScore();
//hide the apple after it reaches the ground by calling this function
hideFallenApple(apple);
}
/**
* Hides the provided element by changing the display property.
* @param {HTMLElement} apple
*/
function hideFallenApple(apple) {
//we need to wait until the apple has fallen down
//so we will use this setTimeout function to wait and the hide the apple
setTimeout(function () {
apple.style.display = 'none';
//call the function that will move the apple to top
//and display it again
restoreFallenApple(apple);
}, 501);
}
/**
* Shows the provided element by changing the display property and restores top position.
* @param {HTMLElement} apple
*/
function restoreFallenApple(apple) {
//as in hideFallenApple we need to wait and the make the html element visible
//restore the top value
apple.style.top = apple.getAttribute('data-top');
setTimeout(function () {
apple.style.display = 'inline-block';
}, 501);
}
/**
* Shows the score in the HTMLElement and checks for High Score.
*
*/
function renderScore() {
scoreBoard.innerText = score;
if (score > highScore) {
highScore = score;
document.getElementById('high').innerText = highScore;
}
}
/**
* Makes the game playable by setting IsPlaying flag to true.
*
*/
function startGame() {
//disable the button to make it unclickable
btnStart.disabled = "disabled";
IsPlaying = true;
renderScore();
//start countDown function and call it every second
//1000 is in millisecond = 1 second
//timer variable stores refrence to the current setInterval
//which will be used to clearInterval later.
timer = setInterval(countDown, 1000);
}
/**
* Performs countDown and the displays the time left.
* if the time has end it will end the game
*
*/
function countDown() {
time = time - 1;
timeBoard.innerText = time;
if (time == 0) {
//clear the interval by using the timer refrence
clearInterval(timer);
//call end game
endGame();
}
}
/**
* Ends the game by setting IsPlaying to false,
* finally resets the score, time and enables btnStart.
*/
function endGame() {
IsPlaying = false;
alert("Your score is " + score);
//reset score and time for next game.
score = 0;
time = 30;
//enable the button to make it clickable
btnStart.removeAttribute('disabled');
}
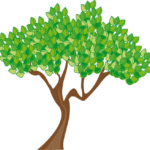
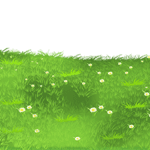
